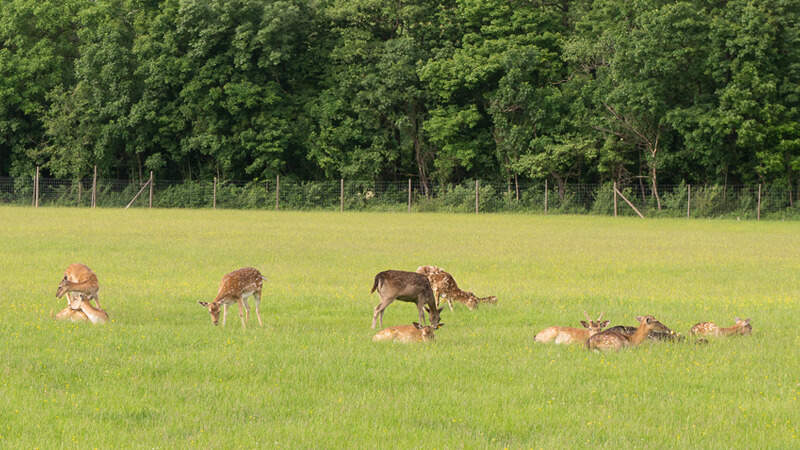
A photo by me on Unsplash
How to create canonical URLs in Gatsby?
📅 18 Jun 2022 | 📖 5 min read
In one of my previous posts I have described what a canonical URL is. Now, let me show you how to implement this in Gatsby.
Content
- Gatsby
- React Helmet
- gatsby-plugin-react-helmet-canonical-urls
- For static pages
- For dynamic templates
- SEO component
- Conclusion
Gatsby
First of all, what is Gatsby?
Gatsby is a React-based open-source framework for creating websites and apps.
Gatsby is a Static Site Generator tool based on React. It takes your React components and pre-renders them into (generates) HTML documents. It has its own way of dealing with page components, dynamic templates, as well as normal reusable components. There is a nice plugin ecosystem and a lively community. If you know React and you want to build fast websites this is a great tool.
After Gatsby renders your components into HTML pages you can serve them as a static website on any hosting you want.
React Helmet
What is React Helmet you might ask. React Helmet is a reusable React component which you can use to manage all of your <head>
tags in your Gatsby project.
This reusable React component will manage all of your changes to the document head. Helmet takes plain HTML tags and outputs plain HTML tags. It's dead simple, and React beginner friendly.
Of course, it is available from the NPM registry. You can use it like this (taken from the official repo for the React Helmet linked above):
import React from 'react';
import { Helmet } from 'react-helmet';
class Application extends React.Component {
render() {
return (
<div className="application">
<Helmet>
<meta charSet="utf-8" />
<title>My Title</title>
<link rel="canonical" href="http://mysite.com/example" />
</Helmet>
...
</div>
);
}
}
Once Gatsby renders the HTML you will get this:
<head>
<meta charset="utf-8" />
<title>My Title</title>
<link rel="canonical" href="http://mysite.com/example" />
</head>
To be able to use this, of course, you have to install some NPM packages:
npm install gatsby-plugin-react-helmet react-helmet
The full installation guide can be found here.
gatsby-plugin-react-helmet-canonical-urls
React Helmet is great, but it did not play well with another Gatsby plugin called gatsby-plugin-canonical-urls
. When using these two combined and you want to override a canonical URL you end up with two canonical meta tags.
That is why the gatsby-plugin-react-helmet-canonical-urls plugin has been created. It is a Gatsby plugin which gives you the possibility to have default canonical meta tags and override them when needed using the React Helmet API.
This is how you use it:
First, install a couple of Gatsby plugins
npm install --save gatsby-plugin-react-helmet gatsby-plugin-react-helmet-canonical-urls
After that add this to your gatsby-config.js
(it is a configuration file for Gatsby)
// In your gatsby-config.js
plugins: [
`gatsby-plugin-react-helmet`,
{
resolve: `gatsby-plugin-react-helmet-canonical-urls`,
options: {
siteUrl: "https://www.example.com",
},
},
]
There are of course some other options you could set up, see the full list of those here.
And that's it. Now, you can use this in your website/application.
For static pages
But, how do you actually use this?
For static pages, you can use something like this:
// in about.jsx for example
export default ({ data, location }) => {
return (
<Layout>
<Helmet>
<title>`${data.site.siteMetadata.title} - About`</title>
<link
rel="canonical"
href={`${data.site.siteMetadata.siteUrl}${location.pathname}`}
/>
</Helmet>
<h2>About me</h2>
</Layout>
);
};
As Gatsby is using GraphQL
you can get that data object filled in while building your pages. Also, you can setup the site
data in your gatsby-config.js
, like this:
// gatsby-config.js
module.exports = {
siteMetadata: {
title: 'Kode Skills',
siteUrl: 'https://kodeskills.com/',
},
};
And then you can access this data in your pages through GraphQL
query, like this:
export const query = graphql`
{
site {
siteMetadata {
title
siteUrl
}
}
}
`;
The location prop is passed to your pages by Gatsby, and you can access useful data with it. In our case, we are using it to access the pathname
, so, basically everything in your URL after the domain name.
For this page it would be /how-to-create-canonical-urls-in-gatsby
.
For dynamic templates
To use this technique for dynamic templates (blog post templates, tags or categories templates, etc...) where one component is reused for dynamic content you can use largely the same approach.
One thing to be aware of is, that you can use data from the frontmatter. This is basically just metadata in your MD files (if you are using those) which you can use to create dynamic canonical URLs.
So, you would have to set this up for your blog posts like this:
// how-to-create-canonical-urls-in-gatsby.md
slug: 'how-to-create-canonical-urls-in-gatsby'
title: 'How to create canonical URLs in Gatsby?'
---
Then in your GraphQL
query for your blog post template:
export const query = graphql`
query BlogPostQuery($slug: String!) {
site {
siteMetadata {
siteUrl
}
}
markdownRemark(frontmatter: { slug: { eq: $slug } }) {
frontmatter {
slug
title
}
}
}
`;
And, finally in your component:
// in blog-post.jsx template
export default ({ data }) => {
return (
<Layout>
<Helmet>
<title>{data.markdownRemark.frontmatter.title}</title>
<link
rel="canonical"
href={`${data.site.siteMetadata.siteUrl}${data.markdownRemark.frontmatter.slug}`}
/>
</Helmet>
... the content of your blog post
</Layout>
);
};
SEO component
To be a bit more flexible and to be able to reuse components for both static and dynamic pages it is a great idea to create a reusable <Seo />
component. Something like this:
// Seo.jsx
export const Seo = ({ canonicalUrl, siteTitle, title }) => {
return (
<>
<Helmet>
<title>
{siteTitle} - {title}
</title>
<link rel="canonical" href={canonicalUrl} />
</Helmet>
</>
);
};
And then you can use it in your templates like this:
// about.jsx - static page template
export default ({ data, location }) => {
return (
<Layout>
<Seo
canonicalUrl={`${data.site.siteMetadata.siteUrl}${location.pathname}`}
siteTitle={data.site.siteMetadata.title}
title="About"
/>
</Layout>
);
};
export const query = graphql`
{
site {
siteMetadata {
title
siteUrl
}
}
}
`;
Or like this in your blog post templates with dynamic data:
// blog-post.jsx
export default ({ data }) => {
return (
<Layout>
<Seo
canonicalUrl={`${data.site.siteMetadata.siteUrl}${data.markdownRemark.frontmatter.slug}`}
siteTitle={data.site.siteMetadata.title}
title={data.markdownRemark.frontmatter.title}
/>
</Layout>
);
};
export const query = graphql`
query BlogPostQuery($slug: String!) {
site {
siteMetadata {
title
siteUrl
}
}
markdownRemark(frontmatter: { slug: { eq: $slug } }) {
frontmatter {
slug
title
}
}
}
`;
Conclusion
Setting up canonical URLs is one of the first things SEO related to my website. It is an important thing to have if you plan to do content syndication, i.e. publishing same content on sites like medium, or dev.to, etc.
I hope this article showed you how easy it is to set this up with a static site generator like Gatsby, which I am using for my website.
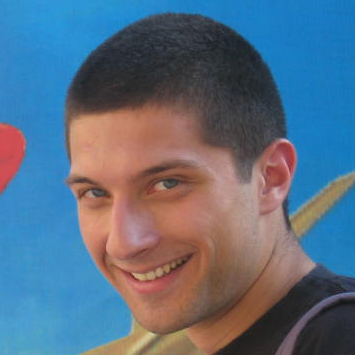
My mission is to empower aspiring developers by making complex web development concepts simple and accessible. Coming from a non-traditional background, I know how challenging it can be to grasp complex topics without formal training. I am dedicated to helping others overcome challenges, launch their careers, and achieve their professional goals in tech.